Have you ever wondered how SVG files render complex text layouts with different styles and directions so seamlessly? At the core of this magic lies text layout algorithms—an essential component of SVG rendering that ensures text appears exactly as intended.
Text layout algorithms are vital for rendering SVGs that include styled or bidirectional text. However, before layout comes text extraction—the process of collecting and organizing text content and properties from the XML tree to enable accurate rendering.
The Extraction Process
SVGs, being XML-based formats, resemble a tree-like structure similar to HTML. To extract information programmatically, you navigate through nodes in this structure.
Each node in the XML tree holds critical details for implementing the SVG2 text layout algorithm, including:
-
- Text content
- Bidi-control properties (manage text directionality)
- Styling attributes like font and spacing
Understanding Bidi-Control
Bidi-control refers to managing text direction (e.g., Left-to-Right or Right-to-Left) using special Unicode characters. This is crucial for accurately displaying mixed-direction text, such as combining English and Arabic.
A Basic Example
<text> foo <tspan>bar</tspan> baz </text>
The diagram and code sample shows the structure librsvg creates when it parses this XML tree.
Here, the <text> element has three children:
-
- A text node containing the characters “foo”.
- A <tspan> element with a single child text node containing “bar”.
- Another text node containing “baz”.
When traversed programmatically, the extracted text from this structure would be “foobarbaz”.
To extract text from the XML tree:
-
- Start traversing nodes from the <text> element.
- Continue through each child until the final closing tag.
- Concatenate character content into a single string.
While this example seems straightforward, real-world SVG2 files introduce additional complexities, such as bidi-control and styling, which must be handled during text extraction.
Handling Complex SVG Trees
Real-world examples often involve more than just plain text nodes. Let’s examine a more complex XML tree that includes styling and bidi-control:
Example:
<text> "Hello" <tspan font-style="bold;">bold</tspan> <tspan direction="rtl" unicode-bidi="bidi-override">مرحبا</tspan> <tspan font-style="italic;">world</tspan> </text>
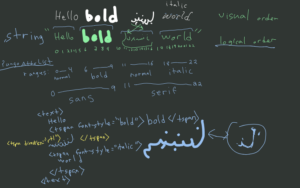
In this example, the <text> element has four children:
-
- A text node containing “Hello”.
- A <tspan> element with font-style: bold, containing the text “bold”.
- A <tspan> element with bidi-control set to RTL (Right-To-Left), containing Arabic text “مرحبا”.
- Another <tspan> element with font-style: italic, containing “world”.
This structure introduces challenges, such as:
-
- Styling: Managing diverse font styles (e.g., bold, italic).
- Whitespace and Positioning: Handling spacing between nodes.
- Bidirectional Control: Ensuring proper text flow for mixed-direction content.
Programmatically extracting text from such structures involves traversing nodes, identifying relevant attributes, and aggregating the text and bidi-control characters accurately.
Why Test-Driven Development Matters
One significant insight during development was the use of Test-Driven Development (TDD), thanks to my mentor Federico. Writing tests before implementation made it easier to visualize and address complex scenarios. This approach turned what initially seemed overwhelming into manageable steps, leading to robust and reliable solutions.
Conclusion
Text extraction is the foundational step in implementing the SVG2 text layout algorithm. By effectively handling complexities such as bidi-control and styling, we ensure that SVGs render text accurately and beautifully, regardless of direction or styling nuances.
If you’ve been following my articles and feel inspired to contribute to librsvg or open source projects, I’d love to hear from you! Drop a comment below to share your thoughts, ask questions, or offer insights. Your contributions—whether in the form of questions, ideas, or suggestions—are invaluable to both the development of librsvg and the ongoing discussion around SVG rendering. 😊
In my next article, we’ll explore how these extracted elements are processed and integrated into the text layout algorithm. Stay tuned—there’s so much more to uncover!