This is one of the final installments on the GtkCellArea TreeView refactoring saga, we’re having a GTK+ team on Tuesday where we’ll discuss this and other various things and finally, land the beast that is GtkCellArea.
Actually as a passing note, the GTK+ team meeting this week is potentially the last one we’re going to have before freezing GTK+ 3.0 apis. If there are glitches, problems, polishing work for GTK+ that you know is important and requires breaking API/ABI, it would be a good idea to present your issues at this meeting.
This is a bit of a surprise post I was not planning on writing but I was surprised by the success of the icon view refactoring work so I thought it would be a good idea to share this here.
Bonus GtkIconView Refactor
I’m pleased to say that the refactored GtkIconView actually works much better than the old icon view, all in ~1700 less lines of code.
A small example of this (without showing off configurable cell alignments and the potential of adding some custom GtkCellArea to your icon view to lay cells out in more custom ways) is already viewable in the tests/testiconview test.
It seems that the former icon view did not handle horizontal layout of cells very well:
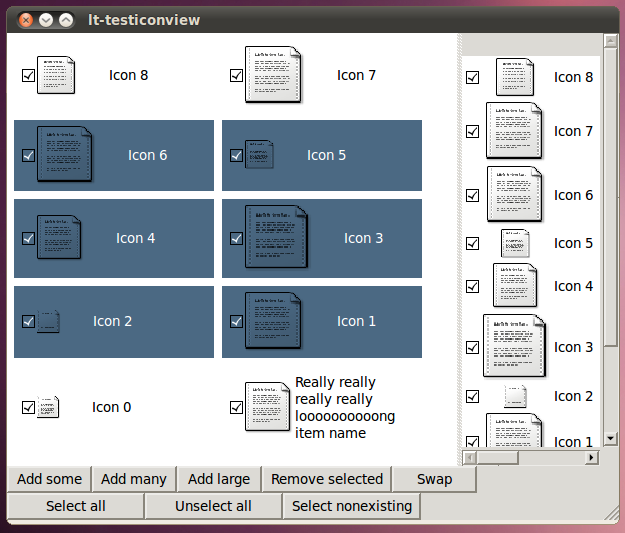
As you can see the cells in this icon view are not aligned, the effect is “yuck”. However this was not noticeable when using a typical GtkIconView with only a constant size pixbuf on the left and variable length text on the right, the “yuck” starts to show through when using variably sized cell renderers on the left followed by more, unaligned renderers.
The new Icon View test in the same configuration:
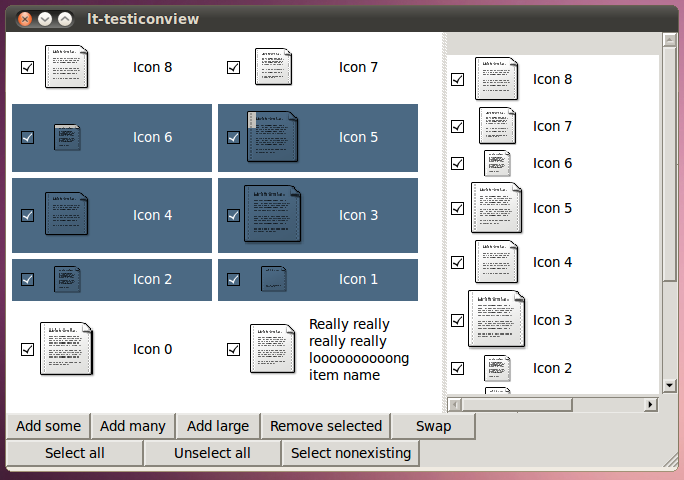
Now all cells are correctly aligned horizontally no matter the size of leading cells.
You’ll have to just trust and forgive me for “Icon 5” and “Icon 6”, it actually does not appear that way. Only when using the screenshot tool and releasing the selected area it causes a rerender of the GtkIconView… it seems the screen capture is performed before the widget has time to finish re-rendering itself.
And that’s not all
The GtkIconView still doesn’t handle progressive calculation of rows like GtkTreeView does (it calculates the sizes of all icons in one go, sorry this refactor did not change that, we still have to take care of that).
However I was curious about the performance difference so I inserted a GTimer into the GtkIconView layouting procedure, which was pretty easy to analyze (since it does everything in one pass, it was pretty easy to compare).
Before refactoring (that is, requesting the size of each GtkCellRenderer individually and doing some complex calculations about where each renderer is placed inside the “icon” or “item”) I got these results:
- After adding 10,000 items to the same test (that’s what the “Add Many” button does), the former icon view took 6.225222 seconds to initially layout the newly added items.
- With the new icon view (after refactoring), adding the same 10,000 rows took 3.492693 seconds.
Once the sizes are initially requested for newly added rows processing is a bit cheaper, however the whole icon view needs to be relayed out every time it gets a new allocation.
With the old code, in my console after resizing the window pane (with > 10,000 items) I got:
relayout took 3.142941 seconds
relayout took 3.150152 seconds
relayout took 3.149025 seconds
With the new code for the same items I got:
relayout took 1.252735 seconds
relayout took 1.339084 seconds
relayout took 1.313939 seconds
relayout took 1.287726 seconds
So in conclusion, not only does the new icon view render cells with better alignments, not only does it do so in 1700 less lines of code… it also calculates the cell positions and lays out the icons in literally half the time it took with the old icon view code.
The icon view refactor work is now ready after ~2 days of work and available on the icon-view-refactor branch.
Enjoy !