It’s been a long time since my last post. After doing the last major UI rework which included a new workflow and the use of a headerbar instead of a menu bar I had little free time to work on the project.
Early this year while on quarantine and in between jobs I started working on things I been wanting to but did not had the time
Fix Glade Survey
On January the GNOME infrastructure was migrated to a new server which broke a small web service running at https://people.gnome.org/~jpu/ used to collect Glade’s survey data.
They also added surveys.gnome.org to conduct any GNOME related surveys making my custom service redundant.
So in order to properly fix the survey I made Glade act like a browser and post the data directly to surveys.gnome.org, no need to open a browser!
This means Glade has to download the survey form, parse it to extract a session token and send it as a cookie for it to work!
JavaScript support
A few years ago while working at Endless Mobile I started adding support for JavaScript widgets since we had several widgets implemented in GJS.
Unfortunately back then a really obscure bug made GJS crash so I never added support for JS in Glade. To my surprise this time around GJS did not crash, at least not on Wayland which led me to find a workaround on X11 and move on
So in order for glade to support your JavaScript widgets you will have to:
- specify gladegjs support code as your plugin library.
- set glade_gjs_init as you init function.
- make sure your catalog name is the same as your JavaScript import library since
glade_gjs_init() will use this name to import your widgets into the
interpreter.
gjsplugin.xml
<glade-catalog name="gjsplugin" library="gladegjs"
domain="glade" depends="gtk+">
<init-function>glade_gjs_init</init-function>
<glade-widget-classes>
<glade-widget-class title="MyJSGrid" name="MyJSGrid"
generic-name="mygrid"/>
</glade-widget-classes>
<glade-widget-group name="gjs" title="Gjs">
<glade-widget-class-ref name="MyJSGrid"/>
</glade-widget-group>
</glade-catalog>
gjsplugin.js
const GObject = imports.gi.GObject;
const Gtk = imports.gi.Gtk;
var MyJSGrid = GObject.registerClass({
GTypeName: 'MyJSGrid',
Properties: {
'string-property': GObject.ParamSpec.string('string-property',
'String Prop',
'Longer description',
GObject.ParamFlags.READWRITE | GObject.ParamFlags.CONSTRUCT,
'Foobar'),
'int-property': GObject.ParamSpec.int('int-property',
'Integer Prop',
'Longer description',
GObject.ParamFlags.READWRITE | GObject.ParamFlags.CONSTRUCT,
0, 10, 5)
},
Signals: {'mysignal': {param_types: [GObject.TYPE_INT]}},
}, class MyJSGrid extends Gtk.Grid {
_init(props) {
super._init(props);
this.label = new Gtk.Label ({ visible: true });
this.add (this.label);
this.connect('notify::string-property',
this._update.bind(this));
this.connect('notify::int-property',
this._update.bind(this));
this._update();
}
_update (obj, pspec) {
this.label.set_text ('JS Properties\nInteger = ' +
this.int_property + '\nString = \'' +
this.string_property + '\'');
}
});
Save these files in a directory added as extra catalog path in the preferences dialog, restart and you should have the new JS classes ready to use.
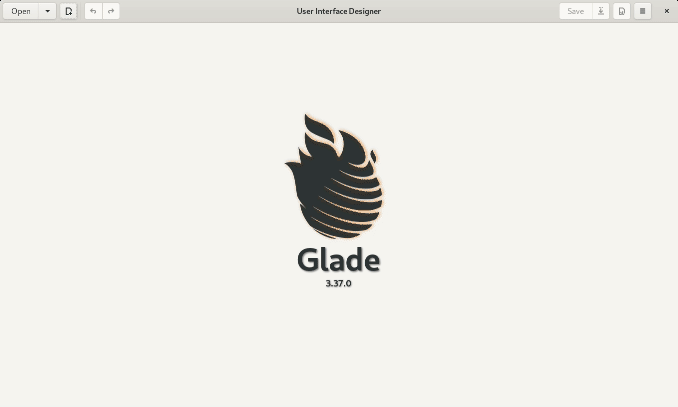
Composite templates improvements
So far the only way to add custom widgets to glade is by writing a catalog which can be tedious so probably not a feature used by most people.
So to make things easier I made Glade load any template file saved in any extra catalog path automatically.
This means all you have to do to is:
- Create your composite template as usual
- Save it on a directory
- Add the directory to the “Extra Catalog & Templates paths” in preferences
- And use your new composite type!
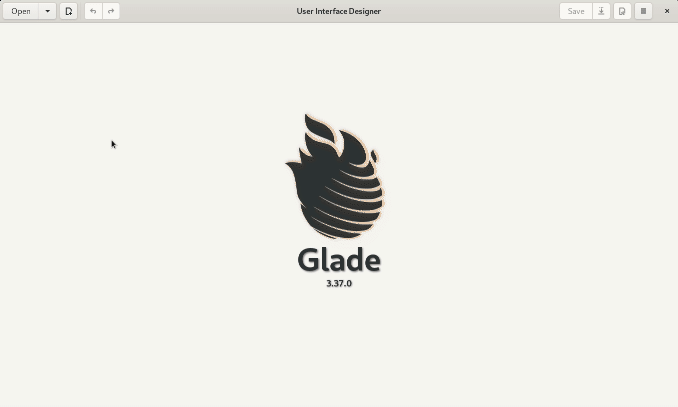
This features will be included in the next stable release, and are already available in nightly flatpak (wiki instructions)
Better deprecations checks
Glade started before GObject introspection so it keeps version and deprecation information in its catalog files which makes it hard to keep it in sync with Gtk. There was also no way to specify in which Gtk version a property or signal was deprecated.
Unfortunately even tough all this metadata is extracted and stored in Gir files it is not available in typelib which makes using libgirepository not an option for Glade instead I decided to make a small script that will take all this information from Gir files and update Glade’s catalog directly making maintaining versioning information way easier!
This means that Glade should catch almost all deprecations and version mismatches even if you are not targeting the latest Gtk version.
Enjoy!
Juan Pablo